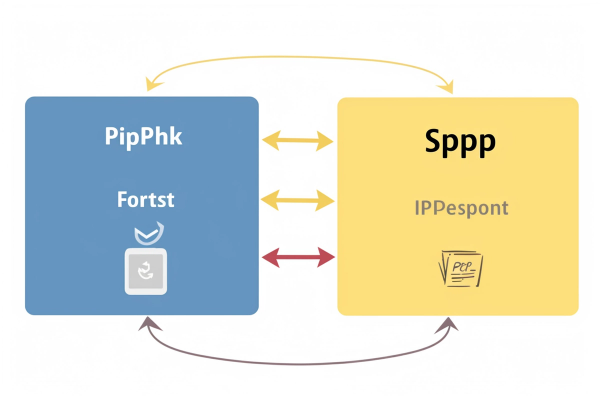
A Comprehensive Comparison: JavaScript vs PHP
Dive into the JavaScript vs PHP showdown! Uncover their syntax, use cases, and performance with hands-on examples. From dynamic front-end magic to robust server-side scripting, witness the power of each language. Choose your coding weapon wisely! 💻✨ #JavaScript #PHP #Coding
Introduction#
When it comes to web development, choosing the right programming language is crucial. JavaScript and PHP are two of the most widely used languages, each with its strengths and weaknesses. In this article, we'll explore and compare various aspects of JavaScript and PHP to help you make an informed decision.
Syntax and Style#
JavaScript#
JavaScript is a versatile scripting language primarily used for front-end development. Its syntax is known for its simplicity and flexibility.
javascriptCopy codefunction greet(name) {
return `Hello, ${name}!`;
}
PHP#
PHP is a server-side scripting language designed for web development. It has a C-style syntax and is embedded within HTML.
phpCopy codefunction greet($name) {
return "Hello, $name!";
}
Use Cases#
JavaScript#
JavaScript is a versatile language used in various contexts:
// Front-end development
const showPopUp = () => {
alert("Welcome to our website!");
};
// Node.js for server-side development
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello from the server!");
});
// Mobile app development with React Native
import React from "react";
import { Text, View } from "react-native";
const App = () => {
return (
<View>
<Text>Hello from React Native!</Text>
</View>
);
};
PHP#
PHP is predominantly used for server-side scripting and web development:
// Server-side scripting
$currentTime = date("H:i:s");
echo "Server time is $currentTime";
// Web development
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>PHP Example</title>
</head>
<body>
<?php
echo "<h1>Hello, PHP!</h1>";
?>
</body>
</html>
// Database integration with MySQL
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "database";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT id, name FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"] . " - Name: " . $row["name"] . "<br>";
}
} else {
echo "0 results";
}
$conn->close();